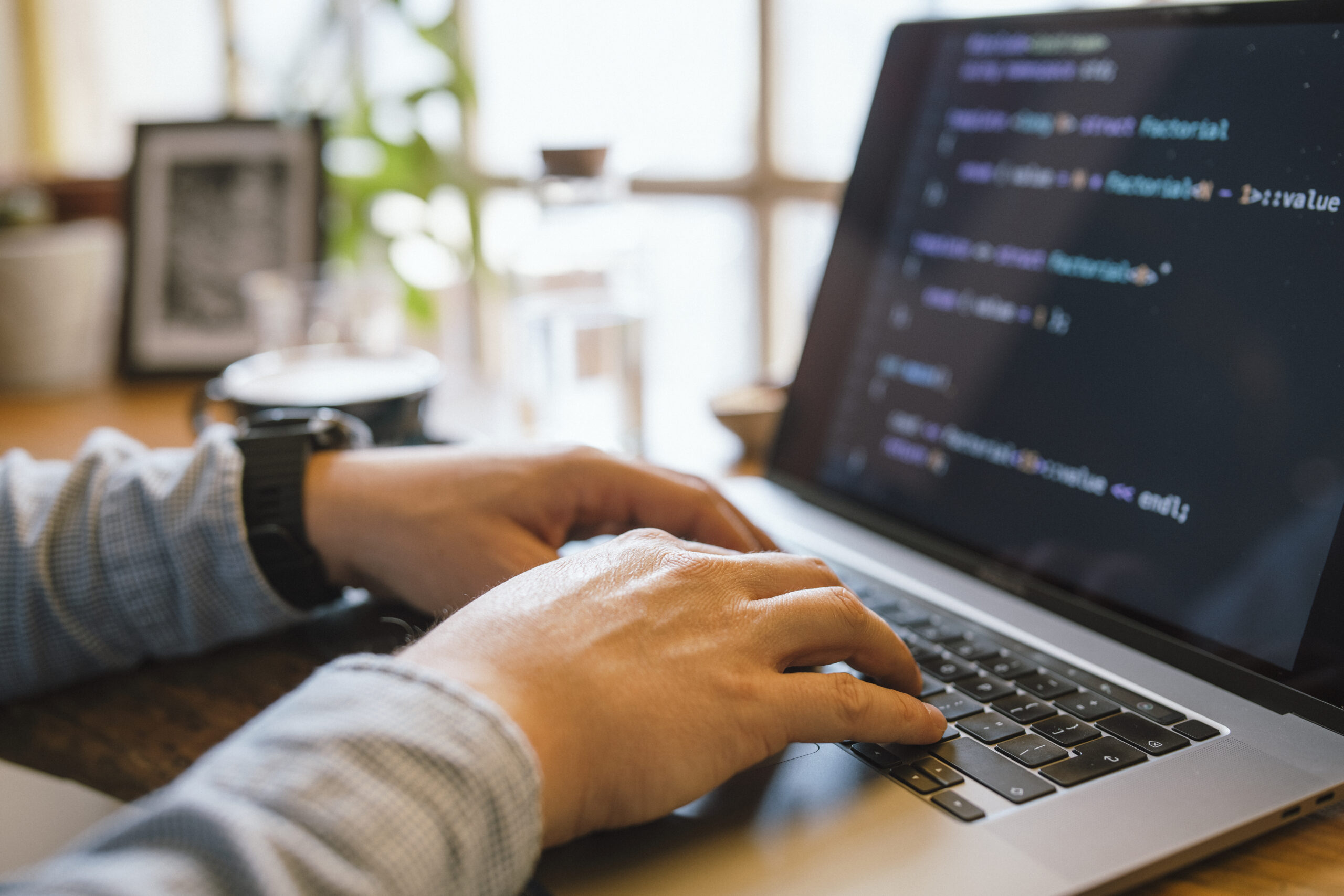
Debugging is One of the more essential — but generally missed — skills inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Feel methodically to solve difficulties effectively. No matter whether you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of stress and substantially increase your productiveness. Listed below are numerous methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is just one Section of improvement, knowing ways to communicate with it efficiently all through execution is Similarly crucial. Modern enhancement environments appear equipped with powerful debugging abilities — but lots of builders only scratch the surface area of what these tools can perform.
Get, for example, an Built-in Advancement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When made use of accurately, they let you observe exactly how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, perspective genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can turn annoying UI challenges into manageable duties.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with Variation Manage techniques like Git to grasp code record, find the exact moment bugs had been launched, and isolate problematic variations.
Ultimately, mastering your tools indicates heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when challenges crop up, you’re not shed in the dark. The greater you know your tools, the greater time you could expend resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most crucial — and often overlooked — steps in helpful debugging is reproducing the condition. In advance of jumping in to the code or making guesses, builders will need to make a steady atmosphere or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact disorders beneath which the bug occurs.
As you’ve collected more than enough details, try to recreate the challenge in your local setting. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider creating automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only support expose the condition but additionally protect against regressions Down the road.
At times, The problem may very well be atmosphere-distinct — it'd happen only on specific running units, browsers, or below certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t only a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools much more successfully, check prospective fixes securely, and talk much more clearly together with your group or customers. It turns an abstract complaint right into a concrete obstacle — Which’s where by builders thrive.
Go through and Realize the Error Messages
Error messages will often be the most beneficial clues a developer has when a little something goes Completely wrong. In lieu of observing them as annoying interruptions, developers should master to take care of error messages as direct communications within the process. They generally let you know precisely what happened, wherever it took place, and occasionally even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. A lot of developers, specially when beneath time pressure, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste mistake messages into search engines like google and yahoo — go through and understand them initially.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it place to a particular file and line range? What module or perform brought on it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can considerably speed up your debugging system.
Some errors are obscure or generic, As well as in those circumstances, it’s important to look at the context by which the error transpired. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A superb logging approach commences with being aware of what to log and at what degree. Frequent logging ranges consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details throughout improvement, INFO for typical gatherings (like prosperous start off-ups), WARN for potential challenges that don’t crack the appliance, ERROR for precise challenges, and FATAL when the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Give attention to important situations, condition alterations, input/output values, and significant selection points in the code.
Structure your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what ailments are satisfied, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with monitoring dashboards.
In the long run, sensible logging is about harmony and clarity. Which has a effectively-considered-out logging approach, it is possible to lessen the time it will take to spot difficulties, acquire deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Think Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To correctly determine and resolve bugs, builders will have to approach the process just like a detective fixing a thriller. This mentality helps break down complicated concerns into workable areas and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Look at the signs of the trouble: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much appropriate facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear picture of what’s happening.
Following, kind hypotheses. Request oneself: What might be creating this behavior? Have any variations a short while ago been designed to your codebase? Has this situation transpired in advance of beneath equivalent conditions? The objective would be to narrow down alternatives and establish probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue inside a managed setting. Should you suspect a specific function or ingredient, isolate it and confirm if the issue persists. Like a detective conducting interviews, ask your code questions and Enable the outcome lead you nearer to the truth.
Pay near consideration to little aspects. Bugs generally conceal in the minimum expected destinations—like a lacking semicolon, an off-by-a single mistake, or even a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential difficulties and assist Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique here complications methodically, and turn out to be simpler at uncovering concealed issues in sophisticated programs.
Generate Tests
Creating exams is among the simplest tips on how to enhance your debugging expertise and Total enhancement performance. Tests not only assist catch bugs early but in addition function a safety Internet that gives you self-assurance when producing alterations on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly exactly where and when a problem occurs.
Start with device checks, which focus on individual capabilities or modules. These compact, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to look, significantly lessening enough time put in debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclusion exams into your workflow. These assist ensure that many portions of your application work jointly easily. They’re particularly handy for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element appropriately, you need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. When the test fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a discouraging guessing activity into a structured and predictable method—serving to you capture more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to become immersed in the situation—gazing your screen for hours, attempting Resolution just after Alternative. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from the new viewpoint.
When you are also near to the code for also extended, cognitive tiredness sets in. You could commence overlooking clear mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind will become considerably less productive at dilemma-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Several developers report getting the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious operate inside the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
In case you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually brings about faster and simpler debugging Ultimately.
In brief, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Every single Bug
Each individual bug you encounter is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful when you go to the trouble to reflect and analyze what went Improper.
Start off by inquiring on your own a handful of key questions once the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The answers often reveal blind places in the workflow or understanding and help you build stronger coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. After some time, you’ll begin to see designs—recurring problems or common issues—you could proactively prevent.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast understanding-sharing session, helping Some others stay away from the same challenge boosts crew performance and cultivates a more robust Studying society.
A lot more importantly, viewing bugs as lessons shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. In fact, several of the best builders are not the ones who generate best code, but those who continually learn from their blunders.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques takes time, follow, and endurance — but the payoff is huge. It would make you a far more efficient, assured, and able developer. Another time you're knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Whatever you do.